こんにちは、タナカです。
この記事では、PyQt5のボタンを作成する関数であるQPushButtonのstylesheetを自分仕様にカスタマイズする方法を説明します。
- QPushButtonのstylesheetデフォルト設定を変更しよう
1. QPushButtonのデフォルト設定
QPushButtonのstylesheetのデフォルト設定は下記のようになっており、カーソルをボタンに合わせると水色に変化します。
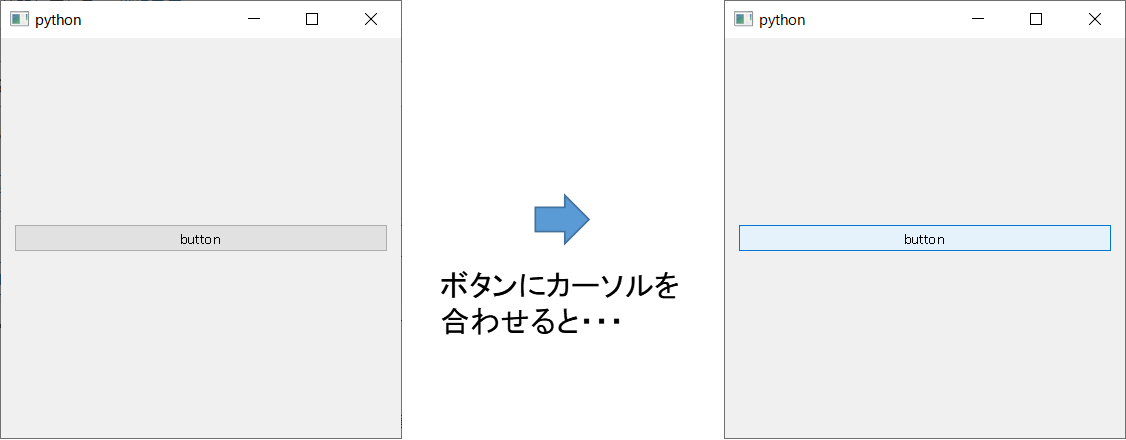
コードはこのようになります。
from PyQt5.QtWidgets import QApplication, QPushButton,QWidget, QHBoxLayout
import sys
class MyWindow(QWidget):
def __init__(self):
super(MyWindow, self).__init__()
self.setGeometry(300, 300, 400, 400)
# layout
self.my_layout = QHBoxLayout()
self.setLayout(self.my_layout)
# button -> layout
self.button = QPushButton('button')
self.my_layout.addWidget(self.button)
def main():
app = QApplication(sys.argv)
win = MyWindow()
win.show()
sys.exit(app.exec_())
main()
このデフォルト設定を自分仕様に変更していきたいと思います。
2. setStyleSheetの活用
setStyleSheetメソッドを使うとQPushButtonのstyleを変更することができます。試しに背景色を赤くしてみます。
self.button.setStyleSheet('QPushButton {background-color: red}')
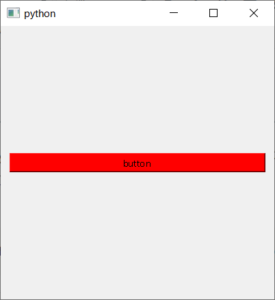
ボタンの背景色が赤色になりました。このとき、先ほどまでは、カーソルをボタンに合わせると水色に変わっていましたが、カーソルをボタンに合わせても色が変わらなくなりました。
styleSheetの設定を変更したことで、デフォルト設定を上書きしたということです。
さらに、ボタンのstyleを変更してみます。
self.button.setStyleSheet('QPushButton {background-color: #4169e1; \
height: 200px; \
color: yellow; \
font: 30px; \
border-radius: 30px;} \
QPushButton:pressed {background: #000080}')
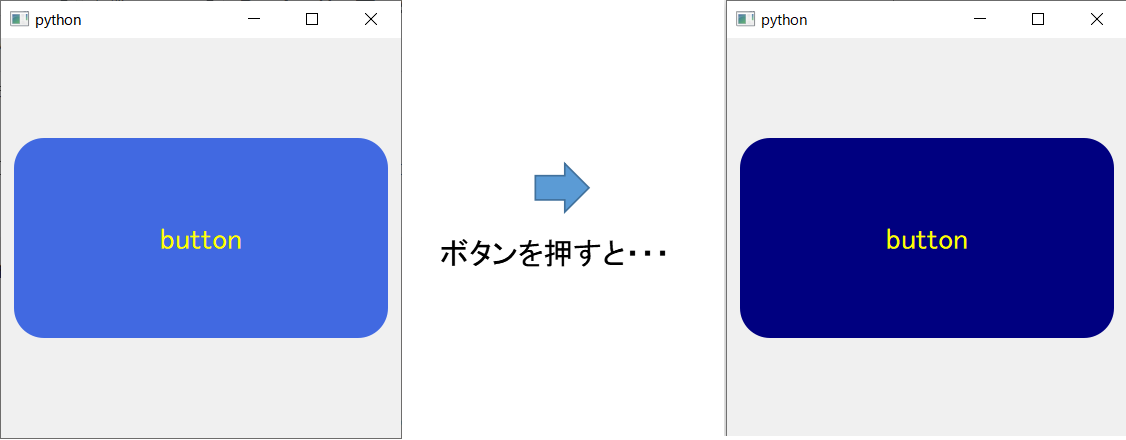
背景色を青、ボタンの高さを200px、フォントの色を黄色、フォントサイズを30px、角を丸くしました。また、ボタンを押した際に、濃い青色に変わるようにしました。
CSSを触ったことがある人は、馴染みがあるのではないでしょうか。
3. 自分のスタイル関数を作る
QPushButtonのstylesheetを自分でカスタマイズできるように関数を作ってみたいと思います。
コードはこのようになります。引数には設定値を入力することで使えるようにしています。
def pushButtonWidgetStyle(self,
height = None, # px
width = None, # px
color = None,
font = None, # px
borderStyle = None,
borderWidth = None, # px
borderColor = None,
borderRadius = None, # px
backgroundColor = None,
hoverBorderStyle = None,
hoverBorderWidth = None, # px
hoverBorderColor = None,
hoverBorderRadius = None, # px
hoverBackgroundColor = None,
pressedBackgroundColor = None,
):
# styleを統合する
widgetStyle = ''
# 基本設定
_height = str.format('height: {};', height)
_width = str.format('width: {};', width)
_color = str.format('color: {};', color)
_font = str.format('font: {};', font)
_borderStyle = str.format('border-style: {};', borderStyle)
_borderWidth = str.format('border-width: {};', borderWidth)
_borderColor = str.format('border-color: {};', borderColor)
_borderRadius = str.format('border-radius: {};', borderRadius)
_backgroundColor = str.format('background-color: {};', backgroundColor)
style = 'QPushButton {%s %s %s %s %s %s %s %s %s}'% (_height, _width, _color, _font, _borderStyle, _borderWidth, _borderColor, _borderRadius, _backgroundColor)
widgetStyle += style
# hover設定
_hoverBorderStyle = str.format('border-style: {};', hoverBorderStyle)
_hoverBorderWidth = str.format('border-width: {};', hoverBorderWidth)
_hoverBorderColor = str.format('border-color: {};', hoverBorderColor)
_hoverBorderRadius = str.format('border-radius: {};', hoverBorderRadius)
_hoverBackgroundColor = str.format('background-color: {};', hoverBackgroundColor)
hoverStyle = 'QPushButton:hover {%s %s %s %s %s}' % (_hoverBorderStyle, _hoverBorderWidth, _hoverBorderColor, _hoverBorderRadius, _hoverBackgroundColor)
widgetStyle += hoverStyle
# pressed設定
_pressedBackgroundColor = str.format('background-color: {};', pressedBackgroundColor)
pressedStyle = 'QPushButton:pressed {%s}' % (_pressedBackgroundColor)
widgetStyle += pressedStyle
return widgetStyle
実際に関数を使ってみます。
self.button_style = self.pushButtonWidgetStyle(height = '100px',
width = '90px',
color = 'red',
font = '30px',
borderStyle = 'solid',
borderWidth = '2px',
borderColor = 'gray',
borderRadius = '6px',
backgroundColor = 'white',
hoverBorderStyle = 'solid',
hoverBorderWidth = '2px',
hoverBorderColor = '#1E90FF',
hoverBorderRadius = '6px',
hoverBackgroundColor = '#98fb98',
pressedBackgroundColor = 'white'
)
self.button.setStyleSheet(self.button_style)
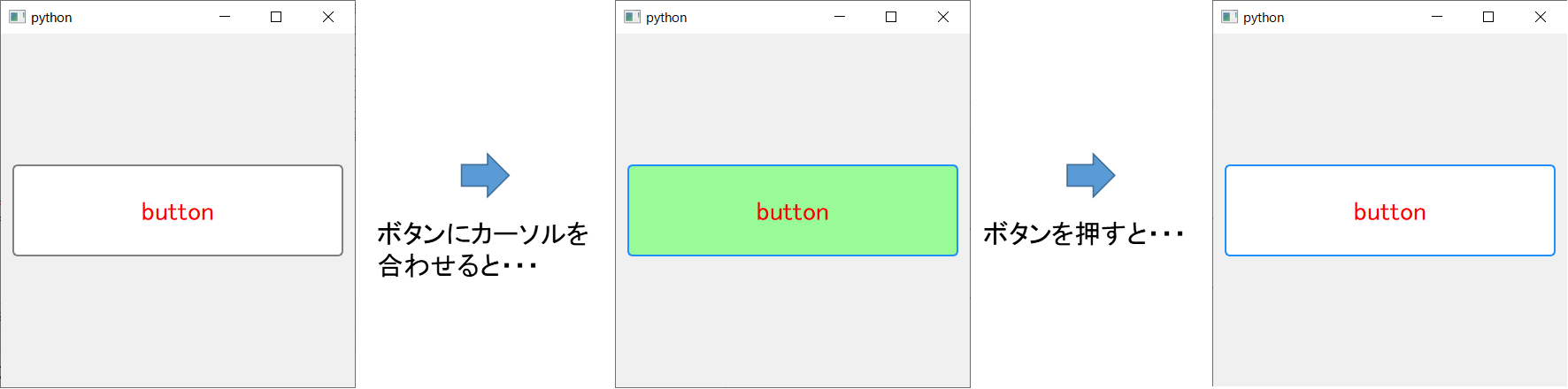
ボタンにカーソルを合わせると黄緑色に変わり、枠の色が水色になるようにしました。さらに、ボタンを押した際に、枠の色のみ水色になるようにしています。
ボタンのstyleSheetを変更することで自分仕様にカスタマイズできることを確認しました。凝ったGUIを作成したい場合は、いろいろカスタマイズしてみると面白いかもしれません。
まとめ
pyqt5のQPushButtonのstylesheetを自分仕様にカスタマイズしてみました。
かなりCSSの書き方に準ずるものがあるので、わかる人はイメージしやすいのではないでしょうか。
今回はQPushButtonについてでしたが、そのほかのWidgetのsytlesheetも同じように変更できるものもあるので、いろいろ試してみるのも楽しいと思います。
最後に、すべてのコードを書いておきます。
from PyQt5.QtWidgets import QApplication, QPushButton,QWidget, QHBoxLayout
import sys
class MyWindow(QWidget):
def __init__(self):
super(MyWindow, self).__init__()
self.setGeometry(300, 300, 400, 400)
self.my_layout = QHBoxLayout()
self.setLayout(self.my_layout)
self.button = QPushButton('button')
self.my_layout.addWidget(self.button)
# self.button.setStyleSheet('QPushButton {background-color: #4169e1; \
# height: 200px; \
# color: yellow; \
# font: 30px; \
# border-radius: 30px;} \
# QPushButton:pressed {background: #000080}')
# self.button.setStyleSheet('QPushButton {background-color: yellow}')
self.button_style = self.pushButtonWidgetStyle(height = '100px',
width = '90px',
color = 'red',
font = '30px',
borderStyle = 'solid',
borderWidth = '2px',
borderColor = 'gray',
borderRadius = '6px',
backgroundColor = 'white',
hoverBorderStyle = 'solid',
hoverBorderWidth = '2px',
hoverBorderColor = '#1E90FF',
hoverBorderRadius = '6px',
hoverBackgroundColor = '#98fb98',
pressedBackgroundColor = 'white'
)
self.button.setStyleSheet(self.button_style)
def pushButtonWidgetStyle(self,
height = None, # px
width = None, # px
color = None,
font = None, # px
borderStyle = None,
borderWidth = None, # px
borderColor = None,
borderRadius = None, # px
backgroundColor = None,
hoverBorderStyle = None,
hoverBorderWidth = None, # px
hoverBorderColor = None,
hoverBorderRadius = None, # px
hoverBackgroundColor = None,
pressedBackgroundColor = None,
):
# styleを統合する
widgetStyle = ''
# 基本設定
_height = str.format('height: {};', height)
_width = str.format('width: {};', width)
_color = str.format('color: {};', color)
_font = str.format('font: {};', font)
_borderStyle = str.format('border-style: {};', borderStyle)
_borderWidth = str.format('border-width: {};', borderWidth)
_borderColor = str.format('border-color: {};', borderColor)
_borderRadius = str.format('border-radius: {};', borderRadius)
_backgroundColor = str.format('background-color: {};', backgroundColor)
style = 'QPushButton {%s %s %s %s %s %s %s %s %s}'% (_height, _width, _color, _font, _borderStyle, _borderWidth, _borderColor, _borderRadius, _backgroundColor)
widgetStyle += style
# hover設定
_hoverBorderStyle = str.format('border-style: {};', hoverBorderStyle)
_hoverBorderWidth = str.format('border-width: {};', hoverBorderWidth)
_hoverBorderColor = str.format('border-color: {};', hoverBorderColor)
_hoverBorderRadius = str.format('border-radius: {};', hoverBorderRadius)
_hoverBackgroundColor = str.format('background-color: {};', hoverBackgroundColor)
hoverStyle = 'QPushButton:hover {%s %s %s %s %s}' % (_hoverBorderStyle, _hoverBorderWidth, _hoverBorderColor, _hoverBorderRadius, _hoverBackgroundColor)
widgetStyle += hoverStyle
# pressed設定
_pressedBackgroundColor = str.format('background-color: {};', pressedBackgroundColor)
pressedStyle = 'QPushButton:pressed {%s}' % (_pressedBackgroundColor)
widgetStyle += pressedStyle
return widgetStyle
def main():
app = QApplication(sys.argv)
win = MyWindow()
win.show()
sys.exit(app.exec_())
main()